Browse other questions tagged json visual-studio-2012 reference package visual-studio-2013 or ask your own question. The Overflow Blog Podcast 332: Non-fungible Talking. Workspace or folder specific tasks are configured from the tasks.json file in the.vscode folder for a workspace. Extensions can also contribute tasks using a Task Provider, and these contributed tasks can add workspace-specific configurations defined in the tasks.json file. To start debugging in Visual Studio Code I have to generate a Launch.json file. After the file has been auto-generated by Visual Studio Code I've got something like this: 'version': '0.2.0', '.

This article shows how to use the System.Text.Json namespace to serialize to and deserialize from JavaScript Object Notation (JSON). If you're porting existing code from Newtonsoft.Json
, see How to migrate to System.Text.Json
.
The directions and sample code use the library directly, not through a framework such as ASP.NET Core.
Most of the serialization sample code sets JsonSerializerOptions.WriteIndented to true
to 'pretty-print' the JSON (with indentation and whitespace for human readability). For production use, you would typically accept the default value of false
for this setting, since adding unnecessary whitespace may incur a noticeable, negative impact on performance and bandwidth usage.
The code examples refer to the following class and variants of it:
Visual Basic support
Parts of System.Text.Json use ref structs, which are not supported by Visual Basic. If you try to use System.Text.Json ref struct APIs with Visual Basic you get BC40000 compiler errors. The error message indicates that the problem is an obsolete API, but the actual issue is lack of ref struct support in the compiler. The following parts of System.Text.Json aren't usable from Visual Basic:
- The Utf8JsonReader class. Since the JsonConverter<T>.Read method takes a
Utf8JsonReader
parameter, this limitation means you can't use Visual Basic to write custom converters. A workaround for this is to implement custom converters in a C# library assembly, and reference that assembly from your VB project. This assumes that all you do in Visual Basic is register the converters into the serializer. You can't call theRead
methods of the converters from Visual Basic code. - Overloads of other APIs that include a ReadOnlySpan<T> type. Most methods include overloads that use
String
instead ofReadOnlySpan
.
These restrictions are in place because ref structs cannot be used safely without language support, even when just 'passing data through.' Subverting this error will result in Visual Basic code that can corrupt memory and should not be done.
Namespaces
The System.Text.Json namespace contains all the entry points and the main types. The System.Text.Json.Serialization namespace contains attributes and APIs for advanced scenarios and customization specific to serialization and deserialization. The code examples shown in this article require using
directives for one or both of these namespaces:
Important
Attributes from the System.Runtime.Serialization namespace aren't supported in System.Text.Json
.
How to write .NET objects as JSON (serialize)
To write JSON to a string or to a file, call the JsonSerializer.Serialize method.
The following example creates JSON as a string:
The following example uses synchronous code to create a JSON file:
The following example uses asynchronous code to create a JSON file:
The preceding examples use type inference for the type being serialized. An overload of Serialize()
takes a generic type parameter:
Serialization example
Here's an example class that contains collection-type properties and a user-defined type:
Tip
'POCO' stands for plain old CLR object. A POCO is a .NET type that doesn't depend on any framework-specific types, for example, through inheritance or attributes.
The JSON output from serializing an instance of the preceding type looks like the following example. The JSON output is minified (whitespace, indentation, and new-line characters are removed) by default:
The following example shows the same JSON, but formatted (that is, pretty-printed with whitespace and indentation):
Serialize to UTF-8
To serialize to UTF-8, call the JsonSerializer.SerializeToUtf8Bytes method:
A Serialize overload that takes a Utf8JsonWriter is also available.
Serializing to UTF-8 is about 5-10% faster than using the string-based methods. The difference is because the bytes (as UTF-8) don't need to be converted to strings (UTF-16).
Serialization behavior
- By default, all public properties are serialized. You can specify properties to ignore.
- The default encoder escapes non-ASCII characters, HTML-sensitive characters within the ASCII-range, and characters that must be escaped according to the RFC 8259 JSON spec.
- By default, JSON is minified. You can pretty-print the JSON.
- By default, casing of JSON names matches the .NET names. You can customize JSON name casing.
- By default, circular references are detected and exceptions thrown. You can preserve references and handle circular references.
- By default, fields are ignored. You can include fields.
When you use System.Text.Json indirectly in an ASP.NET Core app, some default behaviors are different. For more information, see Web defaults for JsonSerializerOptions.
- By default, all public properties are serialized. You can specify properties to ignore.
- The default encoder escapes non-ASCII characters, HTML-sensitive characters within the ASCII-range, and characters that must be escaped according to the RFC 8259 JSON spec.
- By default, JSON is minified. You can pretty-print the JSON.
- By default, casing of JSON names matches the .NET names. You can customize JSON name casing.
- Circular references are detected and exceptions thrown.
- Fields are ignored.
Supported types include:
- .NET primitives that map to JavaScript primitives, such as numeric types, strings, and Boolean.
- User-defined plain old CLR objects (POCOs).
- One-dimensional and jagged arrays (
T[][]
). - Collections and dictionaries from the following namespaces.
- .NET primitives that map to JavaScript primitives, such as numeric types, strings, and Boolean.
- User-defined plain old CLR objects (POCOs).
- One-dimensional and jagged arrays (
ArrayName[][]
). Dictionary<string,TValue>
whereTValue
isobject
,JsonElement
, or a POCO.- Collections from the following namespaces.
You can implement custom converters to handle additional types or to provide functionality that isn't supported by the built-in converters.
How to read JSON as .NET objects (deserialize)
To deserialize from a string or a file, call the JsonSerializer.Deserialize method.
The following example reads JSON from a string and creates an instance of the WeatherForecastWithPOCOs
class shown earlier for the serialization example:
To deserialize from a file by using synchronous code, read the file into a string, as shown in the following example:
To deserialize from a file by using asynchronous code, call the DeserializeAsync method:
Tip
If you have JSON that you want to deserialize, and you don't have the class to deserialize it into, Visual Studio 2019 can automatically generate the class you need:
- Copy the JSON that you need to deserialize.
- Create a class file and delete the template code.
- Choose Edit > Paste Special > Paste JSON as Classes.
The result is a class that you can use for your deserialization target.
Deserialize from UTF-8
To deserialize from UTF-8, call a JsonSerializer.Deserialize overload that takes a ReadOnlySpan<byte>
or a Utf8JsonReader
, as shown in the following examples. The examples assume the JSON is in a byte array named jsonUtf8Bytes.
Deserialization behavior
The following behaviors apply when deserializing JSON:
- By default, property name matching is case-sensitive. You can specify case-insensitivity.
- If the JSON contains a value for a read-only property, the value is ignored and no exception is thrown.
- Non-public constructors are ignored by the serializer.
- Deserialization to immutable objects or read-only properties is supported. See Immutable types and Records.
- By default, enums are supported as numbers. You can serialize enum names as strings.
- By default, fields are ignored. You can include fields.
- By default, comments or trailing commas in the JSON throw exceptions. You can allow comments and trailing commas.
- The default maximum depth is 64.
When you use System.Text.Json indirectly in an ASP.NET Core app, some default behaviors are different. For more information, see Web defaults for JsonSerializerOptions.
- By default, property name matching is case-sensitive. You can specify case-insensitivity. ASP.NET Core apps specify case-insensitivity by default.
- If the JSON contains a value for a read-only property, the value is ignored and no exception is thrown.
- A parameterless constructor, which can be public, internal, or private, is used for deserialization.
- Deserialization to immutable objects or read-only properties isn't supported.
- By default, enums are supported as numbers. You can serialize enum names as strings.
- Fields aren't supported.
- By default, comments or trailing commas in the JSON throw exceptions. You can allow comments and trailing commas.
- The default maximum depth is 64.
When you use System.Text.Json indirectly in an ASP.NET Core app, some default behaviors are different. For more information, see Web defaults for JsonSerializerOptions.
You can implement custom converters to provide functionality that isn't supported by the built-in converters.
Serialize to formatted JSON
To pretty-print the JSON output, set JsonSerializerOptions.WriteIndented to true
:
Here's an example type to be serialized and pretty-printed JSON output:
If you use JsonSerializerOptions
repeatedly with the same options, don't create a new JsonSerializerOptions
instance each time you use it. Reuse the same instance for every call. For more information, see Reuse JsonSerializerOptions instances.
Include fields
Use the JsonSerializerOptions.IncludeFields global setting or the [JsonInclude] attribute to include fields when serializing or deserializing, as shown in the following example:
To ignore read-only fields, use the JsonSerializerOptions.IgnoreReadOnlyFields global setting.
Fields are not supported in System.Text.Json in .NET Core 3.1. Custom converters can provide this functionality.
HttpClient and HttpContent extension methods
Serializing and deserializing JSON payloads from the network are common operations. Extension methods on HttpClient and HttpContent let you do these operations in a single line of code. These extension methods use web defaults for JsonSerializerOptions.
The following example illustrates use of HttpClientJsonExtensions.GetFromJsonAsync and HttpClientJsonExtensions.PostAsJsonAsync:
There are also extension methods for System.Text.Json on HttpContent.
Extension methods on HttpClient
and HttpContent
are not available in System.Text.Json in .NET Core 3.1.
See also
What is jsconfig.json?
The presence of jsconfig.json
file in a directory indicates that the directory is the root of a JavaScript Project. The jsconfig.json
file specifies the root files and the options for the features provided by the JavaScript language service.
Tip: If you are not using JavaScript, you do not need to worry about jsconfig.json
.
Tip:jsconfig.json
is a descendant of tsconfig.json, which is a configuration file for TypeScript. jsconfig.json
is tsconfig.json
with 'allowJs'
attribute set to true
.
Why do I need a jsconfig.json file?
Visual Studio Code's JavaScript support can run in two different modes:
File Scope - no jsconfig.json: In this mode, JavaScript files opened in Visual Studio Code are treated as independent units. As long as a file
a.js
doesn't reference a fileb.ts
explicitly (either usingimport
or CommonJSmodules), there is no common project context between the two files.Explicit Project - with jsconfig.json: A JavaScript project is defined via a
jsconfig.json
file. The presence of such a file in a directory indicates that the directory is the root of a JavaScript project. The file itself can optionally list the files belonging to the project, the files to be excluded from the project, as well as compiler options (see below).
The JavaScript experience is improved when you have a jsconfig.json
file in your workspace that defines the project context. For this reason, we offer a hint to create a jsconfig.json
file when you open a JavaScript file in a fresh workspace.
Location of jsconfig.json
We define this part of our code, the client side of our website, as a JavaScript project by creating a jsconfig.json
file. Place the file at the root of your JavaScript code as shown below.
In more complex projects, you may have more than one jsconfig.json
file defined inside a workspace. You will want to do this so that the code in one project is not suggested as IntelliSense to code in another project. Illustrated below is a project with a client
and server
folder, showing two separate JavaScript projects.
Examples
By default the JavaScript language service will analyze and provide IntelliSense for all files in your JavaScript project. You will want to specify which files to exclude or include in order to provide the proper IntelliSense.
Using the 'exclude'
property
The exclude
attribute (a glob pattern) tells the language service what files are not part of your source code. This keeps performance at a high level. If IntelliSense is slow, add folders to your exclude
list (VS Code will prompt you to do this if it detects the slow down).
Tip: You will want to exclude
files generated by a build process (for example, a dist
directory). These files will cause suggestions to show up twice and will slow down IntelliSense.
Using the 'include'
property
Alternatively, you can explicitly set the files in your project using the include
attribute (a glob pattern). If no include
attribute is present, then this defaults to including all files in the containing directory and subdirectories. When a include
attribute is specified, only those files are included. Here is an example with an explicit include
attribute.
Tip: The file paths in exclude
and include
are relative to the location of jsconfig.json
.
jsconfig Options
Below are jsconfig
'compilerOptions'
to configure the JavaScript language support.
Tip: Do not be confused by compilerOptions
. This attribute exists because jsconfig.json
is a descendant of tsconfig.json
, which is used for compiling TypeScript.
Option | Description |
---|---|
noLib | Do not include the default library file (lib.d.ts) |
target | Specifies which default library (lib.d.ts) to use. The values are 'es3', 'es5', 'es6', 'es2015', 'es2016', 'es2017', 'es2018', 'es2019', 'es2020', 'esnext'. |
module | Specifies the module system, when generating module code. The values are 'amd', 'commonJS', 'es2015', 'es6', 'esnext', 'none', 'system', 'umd'. |
moduleResolution | Specifies how modules are resolved for imports. The values are 'node' and 'classic'. |
checkJs | Enable type checking on JavaScript files. |
experimentalDecorators | Enables experimental support for proposed ES decorators. |
allowSyntheticDefaultImports | Allow default imports from modules with no default export. This does not affect code emit, just type checking. |
baseUrl | Base directory to resolve non-relative module names. |
paths | Specify path mapping to be computed relative to baseUrl option. |
You can read more about the available compilerOptions
in the TypeScript compilerOptions documentation.
Using webpack aliases
For IntelliSense to work with webpack aliases, you need to specify the paths
keys with a glob pattern.
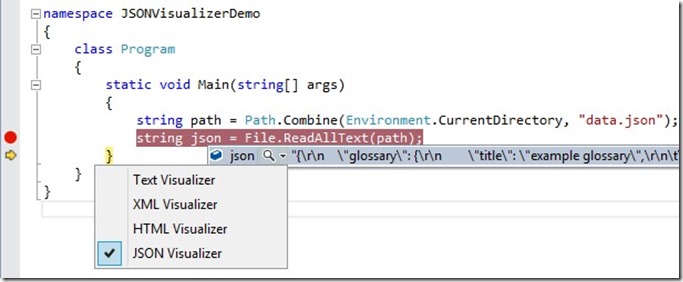
For example, for alias 'ClientApp':
and then to use the alias
Json Reader
Best Practices
Whenever possible, you should exclude folders with JavaScript files that are not part of the source code for your project.
Tip: If you do not have a jsconfig.json
in your workspace, VS Code will by default exclude the node_modules
folder.
Below is a table, mapping common project components to their installation folders that are recommended to exclude:
Visual Studio Json C++
Component | folder to exclude |
---|---|
node | exclude the node_modules folder |
webpack , webpack-dev-server | exclude the content folder, for example dist . |
bower | exclude the bower_components folder |
ember | exclude the tmp and temp folders |
jspm | exclude the jspm_packages folder |
Visual Studio Json Class
When your JavaScript project is growing too large and performance slows, it is often because of library folders like node_modules
. If VS Code detects that your project is growing too large, it will prompt you to edit the exclude
list.
